How to run multiple programs in a sequence using a bash script?
Suppose there are 10 subdirectories inside a parent_directory with the following structure:
***parent_directory (name could be anything - doesn't follow a pattern)
— subdir1 (subdirectory names follow a specific pattern, e.g. fixed characters + number)
input.data (input of the code)
code.f (main code, here is a Fortran code)
Makefile (makefile to execute and run the code)
— subdir2
input files + codes + Makefile
— subdir3
input files + codes + Makefile
.
.
.
— subdir10
Suppose we want to run the codes in the first five subdirectories, i.e. subdir1-subdir5, wait for the processes to finish and then continue running the codes in the next five subdirectories, i.e. subdir6-subdir10.
The example of the bash script is as (download the bash script file
):#!/bin/bash
## exit on the first error.
set -e
# Add the full path of parent_directory to run the codes in the subdirectories
path=$(pwd)
## just for checking the path of the parent_directory
echo path= $path
## the min and the max values of the int variable i, changing from 1 to 5
i=1
imax=5
## do loop from i to imax
while [ $i -le $imax ]; do
## path of each subdirectory defined as "subdir" + integer i
pathi=$path/subdir$i
## just for checking the path of each subdirectory
echo pathi= $pathi
## cd to each subdirectory of the loop
cd $pathi
## compile and run the code in each subdirectory using Makefile ("Main" is the executable file)
make -f Makefile
nohup ./Main &
## adding the counter
let i=i+1
done
## Wait for the processes to finish
wait
i=6
imax=10
## do loop from i to imax
while [ $i -le $imax ]; do
## path of each subdirectory defined as "subdir" + integer i
pathi=$path/subdir$i
## just for checking the path of each subdirectory using Makefile ("Main" is the executable file)
echo pathi= $pathi
## cd to each subdirectory of the loop
cd $pathi
## compile and run the code in each subdirectory
make -f Makefile
nohup ./Main &
## adding the counter
let i=i+1
done
You can run the bash script (called "run.sh") by:
$ bash run.sh
How to copy a file to all subdirectories in a directory using a command-line?
for d in */; do cp code.f "$d"; done
But this will put the file "code.f" in all of the subfolders and their subfolders:
find . -type d -exec cp code.f {} \;
How to delete all files of a specific extension in a directory and all subfolders using a command-line?
find . -name "*.data" -type f -delete
But first use "find" to see exactly which files you will remove:
find . -name "*.data" -type f
Remove file/folder in terminal without asking for permission
Solution: change "rm" command to "\rm"
$ \rm file
$ \rm -r folder
ATOM; a hackable text editor for the 21st Century
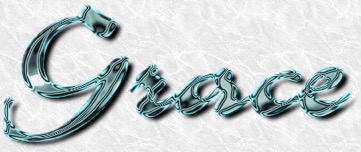
Atom is a text editor that's modern, approachable, yet hackable to the core—a tool you can customize to do anything but also use productively without ever touching a config file.
Download for Mac (For macOS 10.8 or later.)
Other platforms
Beta releases
How do I reload .bashrc without logging out and back in?
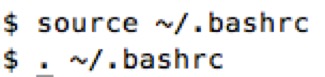
Enter the following command:$ source ~/.bashrc
or$ . ~/.bashrc
Computation Tools: C/Fortran Compilers for Mac OS X
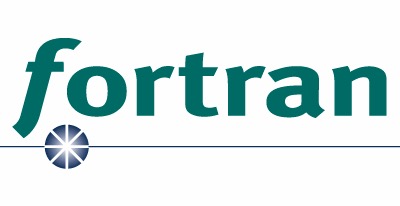
GCC 4.7, 4.8, 4.9 (auto-vectorizing gcc with OpenMP):
Compiled using source code from the GNU servers. This contains current versions (4.7 is the stable release) of gfortran (free, open source, GNU Fortran 95 compiler), gcc (GNU C) and g++ (GNU C++) compilers that can perform auto-vectorization (i.e. modify code to take advantage of AltiVec/SSE, automatically) and other sophisticated optimizations like OpenMP. For more information, see this webpage.
Installation of gfotran:
1) Install Xcode:
You will need to have Apple's XCode Tools installed from the Mac App Store. On LION & M. LION, with XCode 4 or 5 you will need to download the command-line tools as an additional step. You will find the option to download the command-line tools in XCode's Preferences (click on Downloads tab). On 10.9 Mavericks, you can get the command-line tools by simply typing$ xcode-select --install
2) Download one of following binaries,
Binaries:
gcc-4.9-bin.tar.gz, gfortran-4.9-bin.tar.gz (gfortran only), updated Oct 2013 (10.9 Mavericks only).
gcc-4.8-bin.tar.gz, gfortran-4.8-bin.tar.gz (gfortran only), updated Oct 2013 (M. Lion & Mavericks).
gcc-4.7-bin.tar.gz, gfortran-4.7-bin.tar.gz (gfortran only), updated July 2012 (Lion & M. Lion).
Documentation: click here!
3) Installation$ cd to the download folder.
then$ gunzip gcc-4.8-bin.tar.gz
(if your browser didn't do so already)
then$ sudo tar -xvf gcc-lion.tar -C /
It installs everything in /usr/local.
You can invoke the Fortran 95 compiler by simply typing gfortran, as following example:$ gfortran your_program.f
g77 3.4:
This is the FINAL release of g77 (version 3.4 compiler). Future versions of GCC will have gfortran (see above).
Installation of g77:
1) Install Xcode:
You will need to have Apple's XCode Tools installed from the Mac App Store. On LION & M. LION, with XCode 4 or 5 you will need to download the command-line tools as an additional step. You will find the option to download the command-line tools in XCode's Preferences (click on Downloads tab). On 10.9 Mavericks, you can get the command-line tools by simply typing$ xcode-select --install
2) Download one of following binaries
Binaries:
g77-bin.tar.gz (PowerPC only),
g77-intel-bin.tar.gz (Intel Mac only), updated October 2006.
Documentation: click here!
3) Installation$ cd to the download folder.
Then$ gunzip g77-bin.tar.gz
(if your browser didn't do so already)
and$ sudo tar -xvf g77-bin.tar -C /
It installs everything in /usr/local.
F2C based Fortran:
This is the oldest Fortran compiler available for OS X. Its been around since OS X was in a public beta state.
Installation of F2C:
1) Download following shell script:
script: buildf2c
Documentation: click here!
2) then type$ chmod +x buildf2c
and then$ sudo ./buildf2c
The script will grab f2c source from Netlib repositories and install a f2c based compiler in/usr/local/. You are done! The compiler can be envoked by the commands fc or f2c.
Reference: HPC for MAC OS X
Fortran compiler options for full debugging

Intel fortran compiler options for full debugging:
for ifort compiler:
$ ifort -g -traceback -check all -fp-stack-check Program_Name.f -o Program
for F95 compiler:
$ f95 -g -traceback -check all -fp-stack-check Program_Name.f -o Program
*
Program_Name.f
is the name of fortran program.Macbook: how to edit or delete the known_hosts file?
/users/yourusername/.ssh/
to remove the known_hosts file
$ rm known_hosts
to edit the known_hosts file try one of following$ nano known_hosts
$ vim known_hosts
$ vi known_hosts
xdg-open Error: no display specified
When I try to open a URL in the console I get the error message: ERROR: “No Display Specified”
$ xdg-open http://www.google.com
Error: no display specified
Solution
You could try DISPLAY=:0 before xdg-open command$ DISPLAY=:0 xdg-open http://www.google.com
Tips and tricks for optimization of Fortran codes
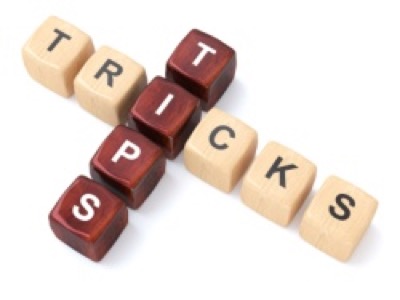
This short report describes the compiler optimization facility and how to use it. It also includes a few examples demonstrating the optimization techniques.
Download the file from HERE (pdf).